#include <windows.h>
#include <stdlib.h>
#include <stdio.h>
#include <math.h>
#include <string.h>
#include "fof_sh.h"
#include "fof_sd.h"
#include "fof_soi.h"
Include dependency graph for fof_soi.c:

Macros | |
#define | e_zmax 0.3 /* {depth of lower boundary, m } */ |
#define | e_Patm 92000 /* {atmospheric pressure at simulation site, Pa} */ |
#define | e_Dvo 2.12e-5 /* {vapor diffusivity in air, m2/s } */ |
#define | e_Tstd 273.15 /* {standard temperature, K } */ |
#define | e_Po 101300 /* {sea level or standard pressure, Pa } */ |
#define | e_R 8.3143 /* {gas constant, J/mol/K } */ |
#define | e_Mw 0.018 /* {mole mass of water, kg/mol } */ |
#define | e_hc 20 /* {surface boundary layer resistance } */ |
#define | e_epse 100 /* {energy balance error - W/m2 } */ |
#define | e_epsw 1e-5 /* {water mass balance error - kg/(m2 s) } */ |
#define | e_dw 1000 /* {density of water - kg/m3 } */ |
#define | e_tor 0.66 /* {soil tortuosity - dimensionless } */ |
#define | e_maxits 20 /* {maximum number of iterations in solution } */ |
#define | e_airvp 1000 /* {air vapor pressure - Pascals } */ |
#define | e_Tair 20 /* {starting air temp - C } */ |
#define | e_lnpo 13.82 /* ln of oven dry water content */ |
Functions | |
REAL | sPOW (REAL x, REAL y) |
int | soiltemp_step (REAL r_Rabs, REAL r_dt, int *ai_success, REAL r_time) |
REAL | tcond (REAL r_t, REAL r_xw, REAL r_xs, REAL r_ls, REAL r_ga, REAL r_xwo, REAL r_cop, REAL r_p, REAL r_s, REAL *ar_enh) |
void | soiltemp_initconsts (REAL r_bdi, REAL r_pdi, REAL r_lsi, REAL r_gai, REAL r_xwoi, REAL r_copi, REAL r_xoi, REAL rr_zi[]) |
void | soiltemp_initprofile (REAL rr_wi[], REAL rr_ti[]) |
REAL | watercontent (REAL r_p, REAL r_xo, REAL *ar_dwdp) |
REAL | humidity (REAL r_p, REAL r_t, REAL *ar_dhdp) |
REAL | vaporpressure (REAL r_tin) |
REAL | slope (REAL r_t, REAL r_p) |
REAL | Hv (REAL r_t) |
REAL | Kvap (REAL r_t, REAL r_p) |
void | soiltemp_getwater (REAL rr_wi[]) |
void | soiltemp_gettemps (REAL rr_ti[]) |
void | soiltemp_getdepths (REAL rr_zi[]) |
REAL | sqr (REAL r) |
REAL | abs_Real (REAL r) |
void | Copy_Array (REAL rr_to[], REAL rr_from[]) |
Variables | |
char | gcr_SoiErr [] |
FILE * | fh_In |
REAL | r_seh |
REAL | r_sev |
REAL | r_wav |
REAL | r_tav |
REAL | r_dJv |
REAL | r_dJvdt |
REAL | r_dJvdp |
REAL | r_bd |
REAL | r_pd |
REAL | r_ls |
REAL | r_ga |
REAL | r_xwo |
REAL | r_cop |
REAL | r_xo |
REAL | r_dC |
REAL | r_dv |
REAL | r_dCdp |
REAL | r_dvdp |
REAL | r_dvdt |
REAL | r_dCdt |
REAL | r_m |
REAL | r_tk |
REAL | r_tk3 |
REAL | r_dtn |
REAL | r_gvol |
REAL | r_ch |
REAL | r_xs |
REAL | r_xws |
REAL | rr_wn [e_mplus1+1] |
REAL | rr_w [e_mplus1+1] |
REAL | rr_z [e_mplus1+1] |
REAL | rr_p [e_mplus1+1] |
REAL | rr_dwdp [e_mplus1+1] |
REAL | rr_v [e_mplus1+1] |
REAL | rr_h [e_mplus1+1] |
REAL | rr_tn [e_mplus1+1] |
REAL | rr_dhdp [e_mplus1+1] |
REAL | rr_psat [e_mplus1+1] |
REAL | rr_kev [e_mplus1+1] |
REAL | rr_u [e_mplus1+1] |
REAL | rr_Hvap [e_mplus1+1] |
REAL | rr_s [e_mplus1+1] |
REAL | rr_ke [e_mplus1+1] |
REAL | rr_kh [e_mplus1+1] |
REAL | rr_kv [e_mplus1+1] |
REAL | rr_cp [e_mplus1+1] |
REAL | rr_conv [e_mplus1+1] |
REAL | rr_vcon [e_mplus1+1] |
REAL | rr_enh [e_mplus1+1] |
REAL | rr_t [e_mplus1+1] |
REAL | rr_AirPor [e_mplus1+1] |
Macro Definition Documentation
#define e_airvp 1000 /* {air vapor pressure - Pascals } */ |
#define e_Dvo 2.12e-5 /* {vapor diffusivity in air, m2/s } */ |
#define e_dw 1000 /* {density of water - kg/m3 } */ |
#define e_epse 100 /* {energy balance error - W/m2 } */ |
#define e_epsw 1e-5 /* {water mass balance error - kg/(m2 s) } */ |
#define e_hc 20 /* {surface boundary layer resistance } */ |
#define e_lnpo 13.82 /* ln of oven dry water content */ |
#define e_maxits 20 /* {maximum number of iterations in solution } */ |
#define e_Mw 0.018 /* {mole mass of water, kg/mol } */ |
#define e_Patm 92000 /* {atmospheric pressure at simulation site, Pa} */ |
#define e_Po 101300 /* {sea level or standard pressure, Pa } */ |
#define e_R 8.3143 /* {gas constant, J/mol/K } */ |
#define e_Tair 20 /* {starting air temp - C } */ |
#define e_tor 0.66 /* {soil tortuosity - dimensionless } */ |
#define e_Tstd 273.15 /* {standard temperature, K } */ |
#define e_zmax 0.3 /* {depth of lower boundary, m } */ |
Function Documentation
Here is the call graph for this function:
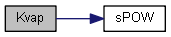
Here is the caller graph for this function:

Here is the call graph for this function:
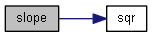
Here is the caller graph for this function:
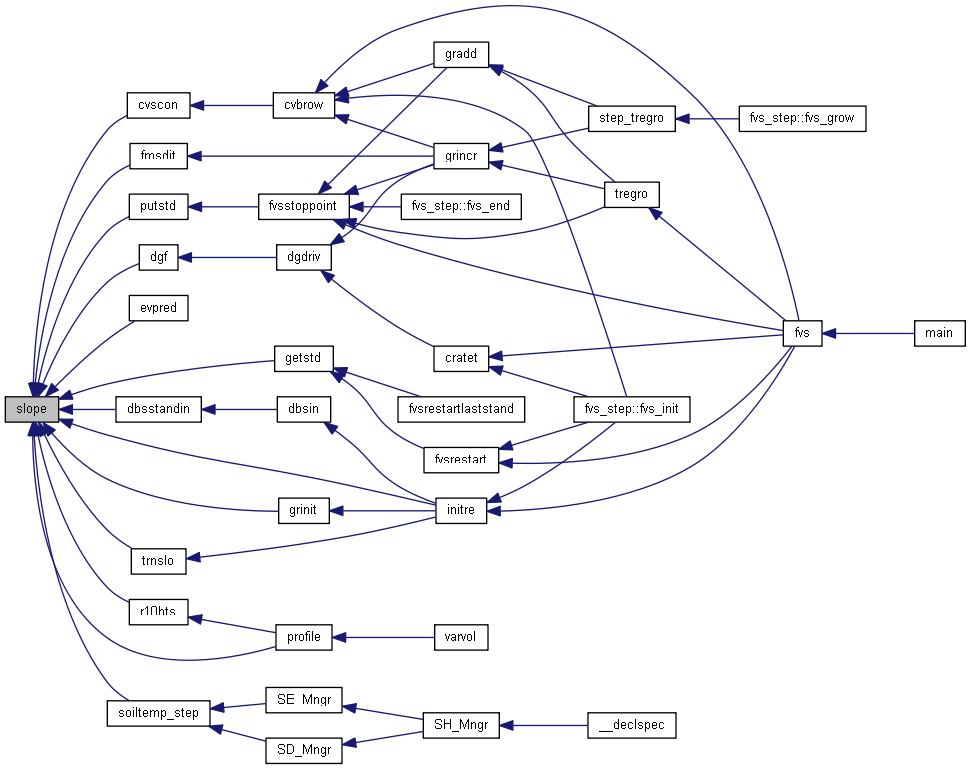
void soiltemp_getdepths | ( | REAL | rr_zi[] | ) |
Here is the call graph for this function:

Here is the caller graph for this function:

void soiltemp_gettemps | ( | REAL | rr_ti[] | ) |
Here is the call graph for this function:

Here is the caller graph for this function:

void soiltemp_getwater | ( | REAL | rr_wi[] | ) |
Here is the call graph for this function:

Here is the caller graph for this function:

void soiltemp_initconsts | ( | REAL | r_bdi, |
REAL | r_pdi, | ||
REAL | r_lsi, | ||
REAL | r_gai, | ||
REAL | r_xwoi, | ||
REAL | r_copi, | ||
REAL | r_xoi, | ||
REAL | rr_zi[] | ||
) |
Here is the caller graph for this function:

Here is the call graph for this function:
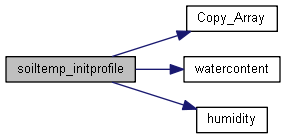
Here is the caller graph for this function:

Here is the call graph for this function:
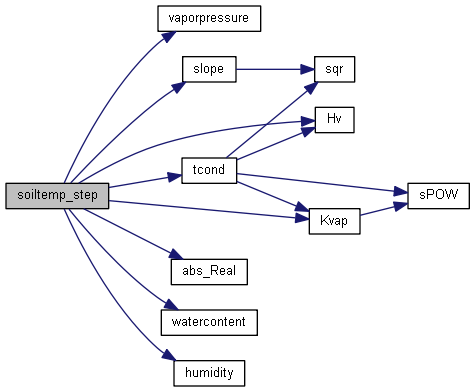
Here is the caller graph for this function:

REAL tcond | ( | REAL | r_t, |
REAL | r_xw, | ||
REAL | r_xs, | ||
REAL | r_ls, | ||
REAL | r_ga, | ||
REAL | r_xwo, | ||
REAL | r_cop, | ||
REAL | r_p, | ||
REAL | r_s, | ||
REAL * | ar_enh | ||
) |
Here is the call graph for this function:
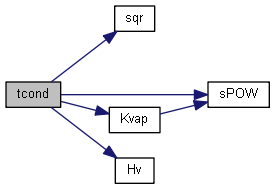
Here is the caller graph for this function:

Here is the caller graph for this function:

Variable Documentation
FILE* fh_In |
char gcr_SoiErr[] |
REAL r_bd |
REAL r_ch |
REAL r_cop |
REAL r_dC |
REAL r_dCdp |
REAL r_dCdt |
REAL r_dJv |
REAL r_dJvdp |
REAL r_dJvdt |
REAL r_dtn |
REAL r_dv |
REAL r_dvdp |
REAL r_dvdt |
REAL r_ga |
REAL r_gvol |
REAL r_ls |
REAL r_m |
REAL r_pd |
REAL r_seh |
REAL r_sev |
REAL r_tav |
REAL r_tk |
REAL r_tk3 |
REAL r_wav |
REAL r_xo |
REAL r_xs |
REAL r_xwo |
REAL r_xws |